如何使用无密码登录链接认证
登录链接,也称为“魔法链接”,是一种无密码认证机制。每当用户想要登录时,都会生成一个新的链接并发送给他们(例如,使用电子邮件)。用户点击链接后,即可在应用程序中完全通过身份验证。
这种身份验证方法可以帮助你消除大多数与身份验证相关的客户支持(例如,我忘记了密码,如何更改或重置密码等)。
使用登录链接认证器
本指南假设你已设置了安全性并在你的应用程序中创建了用户对象。
1) 配置登录链接认证器
登录链接认证器使用防火墙下的 login_link
选项进行配置,并且需要定义两个选项,分别称为 check_route
和 signature_properties
(如下所述)。
1 2 3 4 5 6 7
# config/packages/security.yaml
security:
firewalls:
main:
login_link:
check_route: login_check
signature_properties: ['id']
signature_properties
用于创建签名 URL。这必须至少包含你的 User
对象的一个属性,该属性唯一标识此用户(例如,用户 ID)。阅读更多关于此设置的信息,请参阅下文。
check_route
必须是现有路由的名称,它将用于生成将验证用户身份的登录链接。你不需要控制器(或者它可以为空),因为登录链接认证器将拦截对此路由的请求。
1 2 3 4 5 6 7 8 9 10 11 12 13 14
// src/Controller/SecurityController.php
namespace App\Controller;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\Routing\Attribute\Route;
class SecurityController extends AbstractController
{
#[Route('/login_check', name: 'login_check')]
public function check(): never
{
throw new \LogicException('This code should never be reached');
}
}
2) 生成登录链接
现在认证器能够检查登录链接了,你可以创建一个页面,用户可以在该页面上请求登录链接。
可以使用 LoginLinkHandlerInterface 生成登录链接。当你为这个接口进行类型提示时,正确的登录链接处理器会自动装配给你。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
// src/Controller/SecurityController.php
namespace App\Controller;
use App\Repository\UserRepository;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\HttpFoundation\Response;
use Symfony\Component\Routing\Attribute\Route;
use Symfony\Component\Security\Http\LoginLink\LoginLinkHandlerInterface;
class SecurityController extends AbstractController
{
#[Route('/login', name: 'login')]
public function requestLoginLink(LoginLinkHandlerInterface $loginLinkHandler, UserRepository $userRepository, Request $request): Response
{
// check if form is submitted
if ($request->isMethod('POST')) {
// load the user in some way (e.g. using the form input)
$email = $request->getPayload()->get('email');
$user = $userRepository->findOneBy(['email' => $email]);
// create a login link for $user this returns an instance
// of LoginLinkDetails
$loginLinkDetails = $loginLinkHandler->createLoginLink($user);
$loginLink = $loginLinkDetails->getUrl();
// ... send the link and return a response (see next section)
}
// if it's not submitted, render the form to request the "login link"
return $this->render('security/request_login_link.html.twig');
}
// ...
}
1 2 3 4 5 6 7 8 9
{# templates/security/request_login_link.html.twig #}
{% extends 'base.html.twig' %}
{% block body %}
<form action="{{ path('login') }}" method="POST">
<input type="email" name="email">
<button type="submit">Send Login Link</button>
</form>
{% endblock %}
在此控制器中,用户正在向控制器提交他们的电子邮件地址。基于此属性,加载正确的用户,并使用 createLoginLink() 创建登录链接。
警告
重要的是将此链接发送给用户,而不要直接显示它,因为那样会允许任何人登录。例如,使用 mailer 组件通过邮件将登录链接发送给用户。或者使用该组件向用户的设备发送 SMS。
3) 发送登录链接给用户
现在链接已创建,需要将其发送给用户。任何拥有该链接的人都可以作为该用户登录,因此你需要确保将其发送到他们已知的设备(例如,使用电子邮件或 SMS)。
你可以使用任何库或方法发送链接。但是,登录链接认证器提供了与 Notifier 组件的集成。使用特殊的 LoginLinkNotification 创建通知并将其发送到用户的电子邮件地址或电话号码。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
// src/Controller/SecurityController.php
// ...
use Symfony\Component\Notifier\NotifierInterface;
use Symfony\Component\Notifier\Recipient\Recipient;
use Symfony\Component\Security\Http\LoginLink\LoginLinkNotification;
class SecurityController extends AbstractController
{
#[Route('/login', name: 'login')]
public function requestLoginLink(NotifierInterface $notifier, LoginLinkHandlerInterface $loginLinkHandler, UserRepository $userRepository, Request $request): Response
{
if ($request->isMethod('POST')) {
$email = $request->getPayload()->get('email');
$user = $userRepository->findOneBy(['email' => $email]);
$loginLinkDetails = $loginLinkHandler->createLoginLink($user);
// create a notification based on the login link details
$notification = new LoginLinkNotification(
$loginLinkDetails,
'Welcome to MY WEBSITE!' // email subject
);
// create a recipient for this user
$recipient = new Recipient($user->getEmail());
// send the notification to the user
$notifier->send($notification, $recipient);
// render a "Login link is sent!" page
return $this->render('security/login_link_sent.html.twig');
}
return $this->render('security/request_login_link.html.twig');
}
// ...
}
注意
此集成需要安装和配置 Notifier 和 Mailer 组件。使用以下命令安装所有必需的软件包:
1 2 3
$ composer require symfony/mailer symfony/notifier \
symfony/twig-bundle twig/extra-bundle \
twig/cssinliner-extra twig/inky-extra
这将向用户发送如下电子邮件:
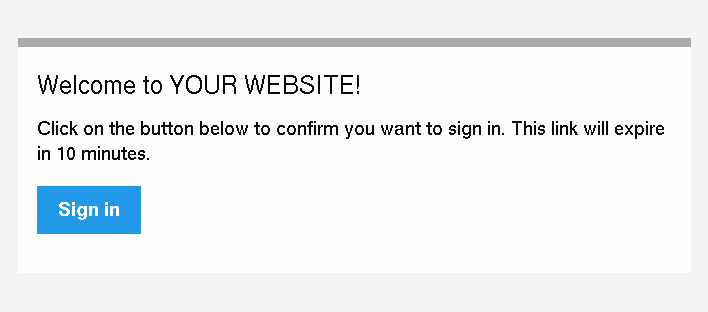
提示
你可以通过扩展 LoginLinkNotification
并配置另一个 htmlTemplate
来自定义此电子邮件模板。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
// src/Notifier/CustomLoginLinkNotification
namespace App\Notifier;
use Symfony\Component\Notifier\Message\EmailMessage;
use Symfony\Component\Notifier\Recipient\EmailRecipientInterface;
use Symfony\Component\Security\Http\LoginLink\LoginLinkNotification;
class CustomLoginLinkNotification extends LoginLinkNotification
{
public function asEmailMessage(EmailRecipientInterface $recipient, ?string $transport = null): ?EmailMessage
{
$emailMessage = parent::asEmailMessage($recipient, $transport);
// get the NotificationEmail object and override the template
$email = $emailMessage->getMessage();
$email->htmlTemplate('emails/custom_login_link_email.html.twig');
return $emailMessage;
}
}
然后,在控制器中使用这个新的 CustomLoginLinkNotification
。
重要注意事项
登录链接是验证用户的便捷方式,但也被认为不如传统的用户名和密码表单安全。不建议在安全关键型应用程序中使用登录链接。
但是,Symfony 中的实现确实有几个扩展点可以使登录链接更安全。在本节中,将讨论最重要的配置决策。
限制登录链接的生命周期
登录链接具有有限的生命周期非常重要。这降低了某人可以拦截链接并使用它以他人身份登录的风险。默认情况下,Symfony 定义了 10 分钟(600 秒)的生命周期。你可以使用 lifetime
选项自定义此设置。
1 2 3 4 5 6 7 8
# config/packages/security.yaml
security:
firewalls:
main:
login_link:
check_route: login_check
# lifetime in seconds
lifetime: 300
提示
你也可以自定义每个链接的生命周期。
使登录链接失效
Symfony 使用签名 URL 来实现登录链接。这样做的好处是,有效的链接不必存储在数据库中。当重要信息发生更改时(例如,用户的电子邮件地址),签名 URL 仍然允许 Symfony 使已发送的登录链接失效。
签名 URL 包含 3 个参数:
expires
- 链接过期的 UNIX 时间戳。
user
- 从此用户的
$user->getUserIdentifier()
返回的值。 hash
expires
、user
和任何已配置签名属性的哈希值。每当这些发生更改时,哈希值也会更改,并且之前的登录链接将失效。
对于在 $user->getUserIdentifier()
调用时返回 user@example.com
的用户,生成的登录链接如下所示:
1
http://example.com/login_check?user=user@example.com&expires=1675707377&hash=f0Jbda56Y...A5sUCI~TQF701fwJ...7m2n4A~
你可以使用 signature_properties
选项向 hash
添加更多属性。
1 2 3 4 5 6 7
# config/packages/security.yaml
security:
firewalls:
main:
login_link:
check_route: login_check
signature_properties: [id, email]
这些属性是使用 PropertyAccess 组件从用户对象中获取的(例如,在本例中使用 getEmail()
或公共 $email
属性)。
提示
你还可以使用签名属性为你的登录链接添加非常高级的失效逻辑。例如,如果你在用户上存储一个 $lastLinkRequestedAt
属性,并在 requestLoginLink()
控制器中更新它,则可以在用户请求新链接时使所有登录链接失效。
配置链接的最大使用次数
限制登录链接的使用次数是登录链接的一个常见特征。Symfony 可以通过在缓存中存储已使用的登录链接来支持此功能。通过设置 max_uses
选项来启用此支持。
1 2 3 4 5 6 7 8 9 10 11
# config/packages/security.yaml
security:
firewalls:
main:
login_link:
check_route: login_check
# only allow the link to be used 3 times
max_uses: 3
# optionally, configure the cache pool
#used_link_cache: 'cache.redis'
确保缓存中有足够的空间,否则无法再存储无效链接(因此会再次变为有效)。过期的无效链接会自动从缓存中删除。
缓存池不会被 cache:clear
命令清除,但是如果缓存组件配置为将缓存存储在该位置,则手动删除 var/cache/
可能会删除缓存。阅读 缓存指南以获取更多信息。
允许链接仅使用一次
当将 max_uses
设置为 1
时,你必须采取额外的预防措施以使其按预期工作。电子邮件提供商和浏览器通常会加载链接的预览,这意味着该链接已被预览加载器失效。
为了解决这个问题,首先设置 check_post_only
选项,让认证器仅处理 HTTP POST 方法。
1 2 3 4 5 6 7 8
# config/packages/security.yaml
security:
firewalls:
main:
login_link:
check_route: login_check
check_post_only: true
max_uses: 1
然后,使用 check_route
控制器来呈现一个页面,该页面允许用户创建此 POST 请求(例如,通过单击按钮)。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
// src/Controller/SecurityController.php
namespace App\Controller;
// ...
use Symfony\Component\Routing\Generator\UrlGeneratorInterface;
class SecurityController extends AbstractController
{
#[Route('/login_check', name: 'login_check')]
public function check(Request $request): Response
{
// get the login link query parameters
$expires = $request->query->get('expires');
$username = $request->query->get('user');
$hash = $request->query->get('hash');
// and render a template with the button
return $this->render('security/process_login_link.html.twig', [
'expires' => $expires,
'user' => $username,
'hash' => $hash,
]);
}
}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
{# templates/security/process_login_link.html.twig #}
{% extends 'base.html.twig' %}
{% block body %}
<h2>Hi! You are about to login to ...</h2>
<!-- for instance, use a form with hidden fields to
create the POST request -->
<form action="{{ path('login_check') }}" method="POST">
<input type="hidden" name="expires" value="{{ expires }}">
<input type="hidden" name="user" value="{{ user }}">
<input type="hidden" name="hash" value="{{ hash }}">
<button type="submit">Continue</button>
</form>
{% endblock %}
哈希策略
在内部,LoginLinkHandler 实现使用 SignatureHasher 来创建登录链接中包含的哈希值。
此哈希器使用链接的到期日期、配置的签名属性的值和用户标识符创建第一个哈希值。使用的哈希算法是 SHA-256。
一旦处理了第一个哈希值并将其编码为 Base64,就会从第一个哈希值和 kernel.secret
容器参数创建一个新的哈希值。这允许 Symfony 对最终哈希值进行签名,该哈希值包含在登录 URL 中。最终哈希值也是一个 Base64 编码的 SHA-256 哈希值。
自定义成功处理器
有时,默认的成功处理不适合你的用例(例如,当你需要生成并返回 API 密钥时)。要自定义成功处理程序的行为方式,请创建你自己的处理程序,作为一个实现 AuthenticationSuccessHandlerInterface 的类。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
// src/Security/Authentication/AuthenticationSuccessHandler.php
namespace App\Security\Authentication;
use Symfony\Component\HttpFoundation\JsonResponse;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\Security\Core\Authentication\Token\TokenInterface;
use Symfony\Component\Security\Http\Authentication\AuthenticationSuccessHandlerInterface;
class AuthenticationSuccessHandler implements AuthenticationSuccessHandlerInterface
{
public function onAuthenticationSuccess(Request $request, TokenInterface $token): JsonResponse
{
$user = $token->getUser();
$userApiToken = $user->getApiToken();
return new JsonResponse(['apiToken' => $userApiToken]);
}
}
然后,将此服务 ID 配置为 success_handler
。
1 2 3 4 5 6 7 8 9
# config/packages/security.yaml
security:
firewalls:
main:
login_link:
check_route: login_check
lifetime: 600
max_uses: 1
success_handler: App\Security\Authentication\AuthenticationSuccessHandler
提示
如果你想自定义默认的失败处理,请使用 failure_handler
选项,并创建一个实现 AuthenticationFailureHandlerInterface 的类。
自定义登录链接
createLoginLink()
方法接受第二个可选参数,以传递生成登录链接时使用的 Request
对象。这允许自定义诸如用于生成链接的语言环境等功能。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
// src/Controller/SecurityController.php
namespace App\Controller;
// ...
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\Security\Http\LoginLink\LoginLinkHandlerInterface;
class SecurityController extends AbstractController
{
#[Route('/login', name: 'login')]
public function requestLoginLink(LoginLinkHandlerInterface $loginLinkHandler, Request $request): Response
{
// check if login form is submitted
if ($request->isMethod('POST')) {
// ... load the user in some way
// clone and customize Request
$userRequest = clone $request;
$userRequest->setLocale($user->getLocale() ?? $request->getDefaultLocale());
// create a login link for $user (this returns an instance of LoginLinkDetails)
$loginLinkDetails = $loginLinkHandler->createLoginLink($user, $userRequest);
$loginLink = $loginLinkDetails->getUrl();
// ...
}
return $this->render('security/request_login_link.html.twig');
}
// ...
}
默认情况下,生成的链接使用全局配置的生命周期,但你可以使用 createLoginLink()
方法的第三个参数更改每个链接的生命周期。
1 2 3
// the third optional argument is the lifetime in seconds
$loginLinkDetails = $loginLinkHandler->createLoginLink($user, null, 60);
$loginLink = $loginLinkDetails->getUrl();