安全
Symfony 提供了许多工具来保护您的应用程序。一些 HTTP 相关的安全工具,例如 安全会话 Cookie 和 CSRF 保护 默认提供。您将在本指南中了解到的 SecurityBundle 提供了保护应用程序所需的所有身份验证和授权功能。
要开始使用,请安装 SecurityBundle
1
$ composer require symfony/security-bundle
如果您安装了 Symfony Flex,这也会为您创建一个 security.yaml
配置文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
# config/packages/security.yaml
security:
# https://symfony.ac.cn/doc/current/security.html#registering-the-user-hashing-passwords
password_hashers:
Symfony\Component\Security\Core\User\PasswordAuthenticatedUserInterface: 'auto'
# https://symfony.ac.cn/doc/current/security.html#where-do-users-come-from-user-providers
providers:
users_in_memory: { memory: null }
firewalls:
dev:
pattern: ^/(_(profiler|wdt)|css|images|js)/
security: false
main:
lazy: true
provider: users_in_memory
# activate different ways to authenticate
# https://symfony.ac.cn/doc/current/security.html#firewalls-authentication
# https://symfony.ac.cn/doc/current/security/impersonating_user.html
# switch_user: true
# Easy way to control access for large sections of your site
# Note: Only the *first* access control that matches will be used
access_control:
# - { path: ^/admin, roles: ROLE_ADMIN }
# - { path: ^/profile, roles: ROLE_USER }
配置内容很多!在接下来的章节中,将讨论三个主要元素
- 用户 (
providers
) - 您应用程序的任何安全部分都需要一些用户概念。用户提供器根据“用户标识符”(例如,用户的电子邮件地址)从任何存储(例如,数据库)加载用户;
- 防火墙 和 验证用户身份 (
firewalls
) - 防火墙是保护应用程序的核心。防火墙内的每个请求都会检查是否需要经过身份验证的用户。防火墙还负责验证此用户的身份(例如,使用登录表单);
- 访问控制(授权) (
access_control
) - 使用访问控制和授权检查器,您可以控制执行特定操作或访问特定 URL 所需的权限。
用户
Symfony 中的权限始终链接到用户对象。如果您需要保护应用程序的(部分)内容,则需要创建一个用户类。这是一个实现 UserInterface 的类。这通常是一个 Doctrine 实体,但您也可以使用专用的 Security 用户类。
生成用户类最简单的方法是使用 MakerBundle 中的 make:user
命令
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
$ php bin/console make:user
The name of the security user class (e.g. User) [User]:
> User
Do you want to store user data in the database (via Doctrine)? (yes/no) [yes]:
> yes
Enter a property name that will be the unique "display" name for the user (e.g. email, username, uuid) [email]:
> email
Will this app need to hash/check user passwords? Choose No if passwords are not needed or will be checked/hashed by some other system (e.g. a single sign-on server).
Does this app need to hash/check user passwords? (yes/no) [yes]:
> yes
created: src/Entity/User.php
created: src/Repository/UserRepository.php
updated: src/Entity/User.php
updated: config/packages/security.yaml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95
// src/Entity/User.php
namespace App\Entity;
use App\Repository\UserRepository;
use Doctrine\ORM\Mapping as ORM;
use Symfony\Component\Security\Core\User\PasswordAuthenticatedUserInterface;
use Symfony\Component\Security\Core\User\UserInterface;
#[ORM\Entity(repositoryClass: UserRepository::class)]
class User implements UserInterface, PasswordAuthenticatedUserInterface
{
#[ORM\Id]
#[ORM\GeneratedValue]
#[ORM\Column(type: 'integer')]
private int $id;
#[ORM\Column(type: 'string', length: 180, unique: true)]
private ?string $email;
#[ORM\Column(type: 'json')]
private array $roles = [];
#[ORM\Column(type: 'string')]
private string $password;
public function getId(): ?int
{
return $this->id;
}
public function getEmail(): ?string
{
return $this->email;
}
public function setEmail(string $email): self
{
$this->email = $email;
return $this;
}
/**
* The public representation of the user (e.g. a username, an email address, etc.)
*
* @see UserInterface
*/
public function getUserIdentifier(): string
{
return (string) $this->email;
}
/**
* @see UserInterface
*/
public function getRoles(): array
{
$roles = $this->roles;
// guarantee every user at least has ROLE_USER
$roles[] = 'ROLE_USER';
return array_unique($roles);
}
public function setRoles(array $roles): self
{
$this->roles = $roles;
return $this;
}
/**
* @see PasswordAuthenticatedUserInterface
*/
public function getPassword(): string
{
return $this->password;
}
public function setPassword(string $password): self
{
$this->password = $password;
return $this;
}
/**
* @see UserInterface
*/
public function eraseCredentials(): void
{
// If you store any temporary, sensitive data on the user, clear it here
// $this->plainPassword = null;
}
}
提示
从 MakerBundle: v1.57.0 开始 - 您可以传递 --with-uuid
或 --with-ulid
给 make:user
。利用 Symfony 的 Uid 组件,这将生成一个 User
实体,其 id
类型为 Uuid 或 Ulid 而不是 int
。
如果您的用户是 Doctrine 实体(如上面的示例),请不要忘记通过 创建和运行迁移 来创建表
1 2
$ php bin/console make:migration
$ php bin/console doctrine:migrations:migrate
提示
从 MakerBundle: v1.56.0 开始 - 将 --formatted
传递给 make:migration
会生成一个美观整洁的迁移文件。
加载用户:用户提供器
除了创建实体外,make:user
命令还在您的安全配置中添加了用户提供器的配置
1 2 3 4 5 6 7 8 9
# config/packages/security.yaml
security:
# ...
providers:
app_user_provider:
entity:
class: App\Entity\User
property: email
此用户提供器知道如何根据“用户标识符”(例如,用户的电子邮件地址或用户名)从存储(例如,数据库)加载(或重新加载)用户。上面的配置使用 Doctrine 加载 User
实体,并将 email
属性用作“用户标识符”。
用户提供器在安全生命周期中的多个位置使用
- 基于标识符加载用户
- 在登录期间(或任何其他身份验证器),提供器根据用户标识符加载用户。一些其他功能,例如 用户模拟 和 记住我 也使用此功能。
- 从会话中重新加载用户
- 在每个请求开始时,用户都会从会话中加载(除非您的防火墙是
stateless
)。提供器“刷新”用户(例如,再次查询数据库以获取最新数据)以确保所有用户信息都是最新的(如有必要,如果发生更改,用户将被取消身份验证/注销)。有关此过程的更多信息,请参阅 安全。
Symfony 附带了几个内置的用户提供器
- 实体用户提供器
- 使用 Doctrine 从数据库加载用户;
- LDAP 用户提供器
- 从 LDAP 服务器加载用户;
- 内存用户提供器
- 从配置文件加载用户;
- 链式用户提供器
- 将两个或多个用户提供器合并为一个新的用户提供器。由于每个防火墙只有一个用户提供器,因此您可以使用它将多个提供器链接在一起。
内置的用户提供器涵盖了应用程序最常见的需求,但您也可以创建自己的 自定义用户提供器。
注意
有时,您需要在另一个类(例如,在您的自定义身份验证器中)中注入用户提供器。所有用户提供器都遵循此模式作为其服务 ID:security.user.provider.concrete.<your-provider-name>
(其中 <your-provider-name>
是配置键,例如 app_user_provider
)。如果您只有一个用户提供器,则可以使用 UserProviderInterface 类型提示自动装配它。
注册用户:哈希密码
许多应用程序需要用户使用密码登录。对于这些应用程序,SecurityBundle 提供了密码哈希和验证功能。
首先,确保您的 User 类实现了 PasswordAuthenticatedUserInterface
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
// src/Entity/User.php
// ...
use Symfony\Component\Security\Core\User\PasswordAuthenticatedUserInterface;
class User implements UserInterface, PasswordAuthenticatedUserInterface
{
// ...
/**
* @return string the hashed password for this user
*/
public function getPassword(): string
{
return $this->password;
}
}
然后,配置应为此类使用哪个密码哈希器。如果您的 security.yaml
文件尚未预先配置,则 make:user
应该为您完成此操作
1 2 3 4 5 6 7
# config/packages/security.yaml
security:
# ...
password_hashers:
# Use native password hasher, which auto-selects and migrates the best
# possible hashing algorithm (which currently is "bcrypt")
Symfony\Component\Security\Core\User\PasswordAuthenticatedUserInterface: 'auto'
现在 Symfony 知道您如何要哈希密码,您可以使用 UserPasswordHasherInterface
服务在将用户保存到数据库之前执行此操作
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
// src/Controller/RegistrationController.php
namespace App\Controller;
// ...
use Symfony\Component\HttpFoundation\Response;
use Symfony\Component\PasswordHasher\Hasher\UserPasswordHasherInterface;
class RegistrationController extends AbstractController
{
public function index(UserPasswordHasherInterface $passwordHasher): Response
{
// ... e.g. get the user data from a registration form
$user = new User(...);
$plaintextPassword = ...;
// hash the password (based on the security.yaml config for the $user class)
$hashedPassword = $passwordHasher->hashPassword(
$user,
$plaintextPassword
);
$user->setPassword($hashedPassword);
// ...
}
}
注意
如果您的用户类是 Doctrine 实体并且您哈希用户密码,则与用户类相关的 Doctrine 存储库类必须实现 PasswordUpgraderInterface。
提示
make:registration-form
maker 命令可以帮助您设置注册控制器并添加诸如使用 SymfonyCastsVerifyEmailBundle 进行电子邮件地址验证之类的功能。
1 2
$ composer require symfonycasts/verify-email-bundle
$ php bin/console make:registration-form
您还可以通过运行以下命令手动哈希密码
1
$ php bin/console security:hash-password
阅读有关 密码哈希和验证 中所有可用哈希器和密码迁移的更多信息。
防火墙
config/packages/security.yaml
的 firewalls
部分是最重要的部分。“防火墙”是您的身份验证系统:防火墙定义了应用程序的哪些部分是安全的,以及您的用户将如何能够进行身份验证(例如,登录表单、API 令牌等)。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
# config/packages/security.yaml
security:
# ...
firewalls:
# the order in which firewalls are defined is very important, as the
# request will be handled by the first firewall whose pattern matches
dev:
pattern: ^/(_(profiler|wdt)|css|images|js)/
security: false
# a firewall with no pattern should be defined last because it will match all requests
main:
lazy: true
# provider that you set earlier inside providers
provider: app_user_provider
# activate different ways to authenticate
# https://symfony.ac.cn/doc/current/security.html#firewalls-authentication
# https://symfony.ac.cn/doc/current/security/impersonating_user.html
# switch_user: true
每个请求只有一个防火墙处于活动状态:Symfony 使用 pattern
键查找第一个匹配项(您也可以 按主机或其他内容匹配)。在这里,所有实际的 URL 都由 main
防火墙处理(没有 pattern
键意味着它匹配所有 URL)。
dev
防火墙实际上是一个虚假的防火墙:它确保您不会意外阻止 Symfony 的开发工具 - 这些工具位于诸如 /_profiler
和 /_wdt
之类的 URL 下。
提示
当匹配多个路由时,您也可以使用更简单的正则表达式数组来匹配每个路由,而不是创建长的正则表达式
1 2 3 4 5 6 7 8 9 10 11 12
# config/packages/security.yaml
security:
# ...
firewalls:
dev:
pattern:
- ^/_profiler/
- ^/_wdt/
- ^/css/
- ^/images/
- ^/js/
# ...
XML 配置文件格式不支持此功能。
所有实际 URL 都由 main
防火墙处理(没有 pattern
键意味着它匹配所有 URL)。防火墙可以有多种身份验证模式,换句话说,它可以启用多种方式来询问“你是谁?”这个问题。
通常,用户在首次访问您的网站时是未知的(即未登录)。如果您现在访问您的主页,您将可以访问,并且您会在工具栏中看到您正在访问防火墙后面的页面
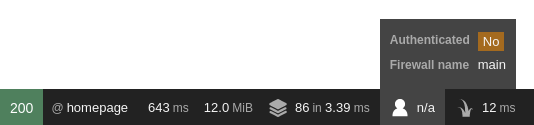
访问防火墙下的 URL 不一定需要您进行身份验证(例如,登录表单必须可访问,或者您的应用程序的某些部分是公共的)。另一方面,您希望感知已登录用户的所有页面都必须在同一防火墙下。因此,如果您想在每个页面上显示“您已登录为...”消息,则所有页面都必须包含在同一防火墙中。
您将在 访问控制 部分了解如何限制对防火墙内的 URL、控制器或任何其他内容的访问。
提示
lazy
匿名模式可防止在不需要授权(即显式检查用户权限)时启动会话。这对于保持请求可缓存非常重要(请参阅 HTTP 缓存)。
为请求获取防火墙配置
如果您需要获取与给定请求匹配的防火墙的配置,请使用 Security 服务
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
// src/Service/ExampleService.php
// ...
use Symfony\Bundle\SecurityBundle\Security;
use Symfony\Component\HttpFoundation\RequestStack;
class ExampleService
{
public function __construct(
// Avoid calling getFirewallConfig() in the constructor: auth may not
// be complete yet. Instead, store the entire Security object.
private Security $security,
private RequestStack $requestStack,
) {
}
public function someMethod(): void
{
$request = $this->requestStack->getCurrentRequest();
$firewallName = $this->security->getFirewallConfig($request)?->getName();
// ...
}
}
验证用户身份
在身份验证期间,系统会尝试为网页访问者找到匹配的用户。传统上,这是使用登录表单或浏览器中的 HTTP basic 对话框完成的。但是,SecurityBundle 附带了许多其他身份验证器
提示
如果您的应用程序通过第三方服务(如 Google、Facebook 或 Twitter(社交登录))登录用户,请查看 HWIOAuthBundle 社区程序包或 Oauth2-client 包。
表单登录
大多数网站都有一个登录表单,用户可以使用标识符(例如,电子邮件地址或用户名)和密码进行身份验证。此功能由内置的 FormLoginAuthenticator 提供。
您可以运行以下命令来创建在应用程序中添加登录表单所需的一切
1
$ php bin/console make:security:form-login
此命令将创建所需的控制器和模板,并且还将更新安全配置。或者,如果您希望手动进行这些更改,请按照后续步骤操作。
首先,为登录表单创建一个控制器
1 2 3 4
$ php bin/console make:controller Login
created: src/Controller/LoginController.php
created: templates/login/index.html.twig
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
// src/Controller/LoginController.php
namespace App\Controller;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Response;
use Symfony\Component\Routing\Attribute\Route;
class LoginController extends AbstractController
{
#[Route('/login', name: 'app_login')]
public function index(): Response
{
return $this->render('login/index.html.twig', [
'controller_name' => 'LoginController',
]);
}
}
然后,使用 form_login
设置启用 FormLoginAuthenticator
1 2 3 4 5 6 7 8 9 10 11
# config/packages/security.yaml
security:
# ...
firewalls:
main:
# ...
form_login:
# "app_login" is the name of the route created previously
login_path: app_login
check_path: app_login
注意
login_path
和 check_path
支持 URL 和路由名称(但不能有强制通配符 - 例如,/login/{foo}
,其中 foo
没有默认值)。
启用后,当未经过身份验证的访问者尝试访问安全位置时,安全系统会将他们重定向到 login_path
(可以使用 身份验证入口点 自定义此行为)。
编辑登录控制器以渲染登录表单
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
// ...
+ use Symfony\Component\Security\Http\Authentication\AuthenticationUtils;
class LoginController extends AbstractController
{
#[Route('/login', name: 'app_login')]
- public function index(): Response
+ public function index(AuthenticationUtils $authenticationUtils): Response
{
+ // get the login error if there is one
+ $error = $authenticationUtils->getLastAuthenticationError();
+
+ // last username entered by the user
+ $lastUsername = $authenticationUtils->getLastUsername();
+
return $this->render('login/index.html.twig', [
- 'controller_name' => 'LoginController',
+ 'last_username' => $lastUsername,
+ 'error' => $error,
]);
}
}
不要让这个控制器让您感到困惑。它的工作只是渲染表单。FormLoginAuthenticator
将自动处理表单提交。如果用户提交了无效的电子邮件或密码,则该身份验证器将存储错误并重定向回此控制器,我们在此控制器中读取错误(使用 AuthenticationUtils
),以便可以将其显示回用户。
最后,创建或更新模板
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
{# templates/login/index.html.twig #}
{% extends 'base.html.twig' %}
{# ... #}
{% block body %}
{% if error %}
<div>{{ error.messageKey|trans(error.messageData, 'security') }}</div>
{% endif %}
<form action="{{ path('app_login') }}" method="post">
<label for="username">Email:</label>
<input type="text" id="username" name="_username" value="{{ last_username }}" required>
<label for="password">Password:</label>
<input type="password" id="password" name="_password" required>
{# If you want to control the URL the user is redirected to on success
<input type="hidden" name="_target_path" value="/account"> #}
<button type="submit">login</button>
</form>
{% endblock %}
警告
传递到模板中的 error
变量是 AuthenticationException 的实例。它可能包含有关身份验证失败的敏感信息。永远不要使用 error.message
:请改用 messageKey
属性,如示例所示。此消息始终可以安全显示。
表单可以看起来像任何样子,但它通常遵循一些约定
<form>
元素向app_login
路由发送POST
请求,因为这是您在security.yaml
中的form_login
键下配置为check_path
的内容;- 用户名(或您用户的“标识符”是什么,例如电子邮件)字段的名称为
_username
,密码字段的名称为_password
。
提示
实际上,所有这些都可以在 form_login
键下配置。有关更多详细信息,请参阅 安全配置参考 (SecurityBundle)。
危险
此登录表单目前未受到 CSRF 攻击的保护。阅读 安全,了解如何保护您的登录表单。
就是这样!当您提交表单时,安全系统会自动读取 _username
和 _password
POST 参数,通过用户提供器加载用户,检查用户的凭据,然后验证用户身份,或者将他们发送回登录表单,并在那里显示错误信息。
回顾整个过程
- 用户尝试访问受保护的资源(例如
/admin
); - 防火墙通过将用户重定向到登录表单(
/login
)来启动身份验证过程; /login
页面通过本示例中创建的路由和控制器渲染登录表单;- 用户将登录表单提交到
/login
; - 安全系统(即
FormLoginAuthenticator
)拦截请求,检查用户提交的凭据,如果凭据正确则验证用户身份,如果凭据不正确则将用户发送回登录表单。
另请参阅
您可以自定义成功或失败登录尝试的响应。请参阅 自定义表单登录验证器响应。
登录表单中的 CSRF 保护
登录 CSRF 攻击 可以使用相同的技术来预防,即在登录表单中添加隐藏的 CSRF 令牌。安全组件已经提供了 CSRF 保护,但您需要配置一些选项才能使用它。
首先,您需要在表单登录中启用 CSRF
1 2 3 4 5 6 7 8 9 10
# config/packages/security.yaml
security:
# ...
firewalls:
secured_area:
# ...
form_login:
# ...
enable_csrf: true
然后,在 Twig 模板中使用 csrf_token()
函数生成 CSRF 令牌,并将其存储为表单的隐藏字段。默认情况下,HTML 字段必须名为 _csrf_token
,用于生成值的字符串必须是 authenticate
1 2 3 4 5 6 7 8 9 10
{# templates/login/index.html.twig #}
{# ... #}
<form action="{{ path('app_login') }}" method="post">
{# ... the login fields #}
<input type="hidden" name="_csrf_token" data-controller="csrf-protection" value="{{ csrf_token('authenticate') }}">
<button type="submit">login</button>
</form>
完成此操作后,您的登录表单就受到了 CSRF 攻击的保护。
提示
您可以通过在配置中设置 csrf_parameter
来更改字段名称,并通过设置 csrf_token_id
来更改令牌 ID。有关更多详细信息,请参阅 安全配置参考 (SecurityBundle)。
JSON 登录
一些应用程序提供使用令牌保护的 API。这些应用程序可能会使用一个端点,该端点基于用户名(或电子邮件)和密码提供这些令牌。JSON 登录验证器可以帮助您创建此功能。
使用 json_login
设置启用验证器
1 2 3 4 5 6 7 8 9 10
# config/packages/security.yaml
security:
# ...
firewalls:
main:
# ...
json_login:
# api_login is a route we will create below
check_path: api_login
注意
check_path
支持 URL 和路由名称(但不能有强制性的通配符 - 例如,/login/{foo}
,其中 foo
没有默认值)。
当客户端请求 check_path
时,验证器运行。首先,为此路径创建一个控制器
1 2 3
$ php bin/console make:controller --no-template ApiLogin
created: src/Controller/ApiLoginController.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
// src/Controller/ApiLoginController.php
namespace App\Controller;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Response;
use Symfony\Component\Routing\Attribute\Route;
class ApiLoginController extends AbstractController
{
#[Route('/api/login', name: 'api_login')]
public function index(): Response
{
return $this->json([
'message' => 'Welcome to your new controller!',
'path' => 'src/Controller/ApiLoginController.php',
]);
}
}
此登录控制器将在验证器成功验证用户身份后被调用。您可以获取已验证的用户,生成令牌(或您需要返回的任何内容),并返回 JSON 响应
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
// ...
+ use App\Entity\User;
+ use Symfony\Component\Security\Http\Attribute\CurrentUser;
class ApiLoginController extends AbstractController
{
- #[Route('/api/login', name: 'api_login')]
+ #[Route('/api/login', name: 'api_login', methods: ['POST'])]
- public function index(): Response
+ public function index(#[CurrentUser] ?User $user): Response
{
+ if (null === $user) {
+ return $this->json([
+ 'message' => 'missing credentials',
+ ], Response::HTTP_UNAUTHORIZED);
+ }
+
+ $token = ...; // somehow create an API token for $user
+
return $this->json([
- 'message' => 'Welcome to your new controller!',
- 'path' => 'src/Controller/ApiLoginController.php',
+ 'user' => $user->getUserIdentifier(),
+ 'token' => $token,
]);
}
}
注意
#[CurrentUser]
只能在控制器参数中使用,以检索已验证的用户。在服务中,您将使用 getUser()。
就是这样!总结一下流程
客户端(例如,前端)向
/api/login
发出带有Content-Type: application/json
标头的 POST 请求,其中包含username
(即使您的标识符实际上是电子邮件)和password
键1 2 3 4
{ "username": "dunglas@example.com", "password": "MyPassword" }
- 安全系统拦截请求,检查用户提交的凭据并验证用户身份。如果凭据不正确,则返回 HTTP 401 Unauthorized JSON 响应,否则将运行您的控制器;
您的控制器创建正确的响应
1 2 3 4
{ "user": "dunglas@example.com", "token": "45be42..." }
提示
JSON 请求格式可以在 json_login
键下配置。有关更多详细信息,请参阅 安全配置参考 (SecurityBundle)。
HTTP Basic 认证
HTTP Basic 身份验证 是一种标准化的 HTTP 身份验证框架。它使用浏览器中的对话框询问凭据(用户名和密码),Symfony 的 HTTP basic 验证器将验证这些凭据。
将 http_basic
键添加到您的防火墙以启用 HTTP Basic 身份验证
1 2 3 4 5 6 7 8 9
# config/packages/security.yaml
security:
# ...
firewalls:
main:
# ...
http_basic:
realm: Secured Area
就是这样!每当未经身份验证的用户尝试访问受保护的页面时,Symfony 都会通知浏览器需要启动 HTTP basic 身份验证(使用 WWW-Authenticate
响应头)。然后,验证器验证凭据并验证用户身份。
注意
您不能将 注销 与 HTTP basic 验证器一起使用。即使您从 Symfony 注销,您的浏览器也会“记住”您的凭据,并在每次请求时发送它们。
X.509 客户端证书
当使用客户端证书时,您的 Web 服务器会自行完成所有身份验证。Symfony 提供的 X.509 验证器从客户端证书的“专有名称”(DN)中提取电子邮件。然后,它在用户提供器中使用此电子邮件作为用户标识符。
首先,配置您的 Web 服务器以启用客户端证书验证,并将证书的 DN 暴露给 Symfony 应用程序
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
server {
# ...
ssl_client_certificate /path/to/my-custom-CA.pem;
# enable client certificate verification
ssl_verify_client optional;
ssl_verify_depth 1;
location / {
# pass the DN as "SSL_CLIENT_S_DN" to the application
fastcgi_param SSL_CLIENT_S_DN $ssl_client_s_dn;
# ...
}
}
然后,在您的防火墙上使用 x509
启用 X.509 验证器
1 2 3 4 5 6 7 8 9
# config/packages/security.yaml
security:
# ...
firewalls:
main:
# ...
x509:
provider: your_user_provider
默认情况下,Symfony 以两种不同的方式从 DN 中提取电子邮件地址
- 首先,它尝试
SSL_CLIENT_S_DN_Email
服务器参数,该参数由 Apache 暴露; - 如果未设置(例如,当使用 Nginx 时),它将使用
SSL_CLIENT_S_DN
并匹配emailAddress
后面的值。
您可以在 x509
键下自定义某些参数的名称。有关更多详细信息,请参阅 x509 配置参考。
远程用户
除了客户端证书身份验证之外,还有更多 Web 服务器模块可以预先验证用户身份(例如 kerberos)。远程用户验证器为这些服务提供了基本集成。
这些模块通常在 REMOTE_USER
环境变量中暴露已验证的用户。远程用户验证器使用此值作为用户标识符来加载相应的用户。
使用 remote_user
键启用远程用户身份验证
1 2 3 4 5 6 7
# config/packages/security.yaml
security:
firewalls:
main:
# ...
remote_user:
provider: your_user_provider
提示
您可以在 remote_user
键下自定义此服务器变量的名称。有关更多详细信息,请参阅 配置参考。
限制登录尝试次数
Symfony 借助 速率限制器组件,提供了针对 暴力破解登录攻击 的基本保护。如果您尚未在应用程序中使用此组件,请在使用此功能之前安装它
1
$ composer require symfony/rate-limiter
然后,使用 login_throttling
设置启用此功能
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
# config/packages/security.yaml
security:
firewalls:
# ...
main:
# ...
# by default, the feature allows 5 login attempts per minute
login_throttling: null
# configure the maximum login attempts
login_throttling:
max_attempts: 3 # per minute ...
# interval: '15 minutes' # ... or in a custom period
# use a custom rate limiter via its service ID
login_throttling:
limiter: app.my_login_rate_limiter
注意
interval
选项的值必须是一个数字,后跟 PHP 日期相对格式 接受的任何单位(例如 3 seconds
、10 hours
、1 day
等)
在内部,Symfony 使用 速率限制器组件,该组件默认使用 Symfony 的缓存来存储之前的登录尝试。但是,您可以实现 自定义存储。
登录尝试在 max_attempts
(默认值:5)次失败请求后,对 IP 地址 + 用户名
和 IP 地址
的 5 * max_attempts
次失败请求进行限制。第二个限制防止攻击者使用多个用户名绕过第一个限制,而不会扰乱大型网络(如办公室)上的正常用户。
提示
限制失败的登录尝试只是针对暴力破解攻击的基本保护之一。OWASP 暴力破解攻击 指南提到了您应该根据所需保护级别考虑的其他几种保护措施。
如果您需要更复杂的限制算法,请创建一个实现 RequestRateLimiterInterface 的类(或使用 DefaultLoginRateLimiter),并将 limiter
选项设置为其服务 ID
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
# config/packages/security.yaml
framework:
rate_limiter:
# define 2 rate limiters (one for username+IP, the other for IP)
username_ip_login:
policy: token_bucket
limit: 5
rate: { interval: '5 minutes' }
ip_login:
policy: sliding_window
limit: 50
interval: '15 minutes'
services:
# our custom login rate limiter
app.login_rate_limiter:
class: Symfony\Component\Security\Http\RateLimiter\DefaultLoginRateLimiter
arguments:
# globalFactory is the limiter for IP
$globalFactory: '@limiter.ip_login'
# localFactory is the limiter for username+IP
$localFactory: '@limiter.username_ip_login'
$secret: '%kernel.secret%'
security:
firewalls:
main:
# use a custom rate limiter via its service ID
login_throttling:
limiter: app.login_rate_limiter
自定义成功和失败的身份验证行为
如果您想自定义成功或失败的身份验证过程的处理方式,您不必全局覆盖相应的监听器。相反,您可以通过实现 AuthenticationSuccessHandlerInterface 或 AuthenticationFailureHandlerInterface 来设置自定义的成功失败处理程序。
阅读 如何自定义您的成功处理程序,以获取有关此的更多信息。
以编程方式登录
您可以使用 Security 助手的 login()
方法以编程方式登录用户
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
// src/Controller/SecurityController.php
namespace App\Controller;
use App\Security\Authenticator\ExampleAuthenticator;
use Symfony\Bundle\SecurityBundle\Security;
use Symfony\Component\Security\Http\Authenticator\Passport\Badge\RememberMeBadge;
class SecurityController
{
public function someAction(Security $security): Response
{
// get the user to be authenticated
$user = ...;
// log the user in on the current firewall
$security->login($user);
// if the firewall has more than one authenticator, you must pass it explicitly
// by using the name of built-in authenticators...
$security->login($user, 'form_login');
// ...or the service id of custom authenticators
$security->login($user, ExampleAuthenticator::class);
// you can also log in on a different firewall...
$security->login($user, 'form_login', 'other_firewall');
// ... add badges...
$security->login($user, 'form_login', 'other_firewall', [(new RememberMeBadge())->enable()]);
// ... and also add passport attributes
$security->login($user, 'form_login', 'other_firewall', [(new RememberMeBadge())->enable()], ['referer' => 'https://oauth.example.com']);
// use the redirection logic applied to regular login
$redirectResponse = $security->login($user);
return $redirectResponse;
// or use a custom redirection logic (e.g. redirect users to their account page)
// return new RedirectResponse('...');
}
}
7.2
login() 方法中对 passport 属性的支持是在 Symfony 7.2 中引入的。
注销
要启用注销,请在您的防火墙下激活 logout
配置参数
1 2 3 4 5 6 7 8 9 10 11 12
# config/packages/security.yaml
security:
# ...
firewalls:
main:
# ...
logout:
path: /logout
# where to redirect after logout
# target: app_any_route
然后,Symfony 将取消对导航到配置的 path
的用户的身份验证,并将他们重定向到配置的 target
。
提示
如果您需要引用注销路径,可以使用 _logout_<firewallname>
路由名称(例如 _logout_main
)。
如果您的项目未使用 Symfony Flex,请确保您已在路由中导入了注销路由加载器
1 2 3 4
# config/routes/security.yaml
_symfony_logout:
resource: security.route_loader.logout
type: service
以编程方式注销
您可以使用 Security 助手的 logout()
方法以编程方式注销用户
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
// src/Controller/SecurityController.php
namespace App\Controller\SecurityController;
use Symfony\Bundle\SecurityBundle\Security;
class SecurityController
{
public function someAction(Security $security): Response
{
// logout the user in on the current firewall
$response = $security->logout();
// you can also disable the csrf logout
$response = $security->logout(false);
// ... return $response (if set) or e.g. redirect to the homepage
}
}
用户将从请求的防火墙中注销。如果请求不在防火墙后面,则会抛出 \LogicException
。
自定义注销
在某些情况下,您需要在注销时运行额外的逻辑(例如,使某些令牌失效)或想要自定义注销后发生的事情。在注销期间,会分发 LogoutEvent。注册一个 事件监听器或订阅者 以执行自定义逻辑
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
// src/EventListener/LogoutSubscriber.php
namespace App\EventListener;
use Symfony\Component\EventDispatcher\EventSubscriberInterface;
use Symfony\Component\HttpFoundation\RedirectResponse;
use Symfony\Component\Routing\Generator\UrlGeneratorInterface;
use Symfony\Component\Security\Http\Event\LogoutEvent;
class LogoutSubscriber implements EventSubscriberInterface
{
public function __construct(
private UrlGeneratorInterface $urlGenerator
) {
}
public static function getSubscribedEvents(): array
{
return [LogoutEvent::class => 'onLogout'];
}
public function onLogout(LogoutEvent $event): void
{
// get the security token of the session that is about to be logged out
$token = $event->getToken();
// get the current request
$request = $event->getRequest();
// get the current response, if it is already set by another listener
$response = $event->getResponse();
// configure a custom logout response to the homepage
$response = new RedirectResponse(
$this->urlGenerator->generate('homepage'),
RedirectResponse::HTTP_SEE_OTHER
);
$event->setResponse($response);
}
}
自定义注销路径
另一个选项是将 path
配置为路由名称。如果您希望注销 URI 是动态的(例如,根据当前区域设置进行翻译),这将非常有用。在这种情况下,您必须自己创建此路由
1 2 3 4 5 6
# config/routes.yaml
app_logout:
path:
en: /logout
fr: /deconnexion
methods: GET
然后,将路由名称传递给 path
选项
1 2 3 4 5 6 7 8 9
# config/packages/security.yaml
security:
# ...
firewalls:
main:
# ...
logout:
path: app_logout
获取用户对象
身份验证后,可以通过 基础控制器 中的 getUser()
快捷方式访问当前用户的 User
对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Response;
class ProfileController extends AbstractController
{
public function index(): Response
{
// usually you'll want to make sure the user is authenticated first,
// see "Authorization" below
$this->denyAccessUnlessGranted('IS_AUTHENTICATED_FULLY');
// returns your User object, or null if the user is not authenticated
// use inline documentation to tell your editor your exact User class
/** @var \App\Entity\User $user */
$user = $this->getUser();
// Call whatever methods you've added to your User class
// For example, if you added a getFirstName() method, you can use that.
return new Response('Well hi there '.$user->getFirstName());
}
}
从服务中获取用户
如果您需要从服务中获取已登录用户,请使用 Security 服务
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
// src/Service/ExampleService.php
// ...
use Symfony\Bundle\SecurityBundle\Security;
class ExampleService
{
// Avoid calling getUser() in the constructor: auth may not
// be complete yet. Instead, store the entire Security object.
public function __construct(
private Security $security,
){
}
public function someMethod(): void
{
// returns User object or null if not authenticated
$user = $this->security->getUser();
// ...
}
}
在模板中获取用户
在 Twig 模板中,用户对象可以通过 Twig 全局 app 变量 的 app.user
变量获得
1 2 3
{% if is_granted('IS_AUTHENTICATED_FULLY') %}
<p>Email: {{ app.user.email }}</p>
{% endif %}
访问控制(授权)
用户现在可以使用您的登录表单登录您的应用。太棒了!现在,您需要学习如何拒绝访问以及如何使用 User 对象。这称为授权,其工作是决定用户是否可以访问某些资源(URL、模型对象、方法调用等)。
授权过程有两个不同的方面
- 用户在登录时收到特定的角色(例如
ROLE_ADMIN
)。 - 您添加代码,以便资源(例如 URL、控制器)需要特定的“属性”(例如像
ROLE_ADMIN
这样的角色)才能被访问。
角色
当用户登录时,Symfony 会在您的 User
对象上调用 getRoles()
方法,以确定该用户具有哪些角色。在之前生成的 User
类中,角色是一个存储在数据库中的数组,并且每个用户始终至少被赋予一个角色:ROLE_USER
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
// src/Entity/User.php
// ...
class User
{
#[ORM\Column(type: 'json')]
private array $roles = [];
// ...
public function getRoles(): array
{
$roles = $this->roles;
// guarantee every user at least has ROLE_USER
$roles[] = 'ROLE_USER';
return array_unique($roles);
}
}
这是一个不错的默认设置,但您可以随意决定用户应具有哪些角色。唯一的规则是每个角色必须以 ROLE_
前缀开头 - 否则,事情将无法按预期工作。除此之外,角色只是一个字符串,您可以发明任何您需要的东西(例如 ROLE_PRODUCT_ADMIN
)。
接下来您将使用这些角色来授予对您网站特定部分的访问权限。
分层角色
您可以创建角色层次结构来定义角色继承规则,而不是为每个用户分配许多角色
1 2 3 4 5 6 7
# config/packages/security.yaml
security:
# ...
role_hierarchy:
ROLE_ADMIN: ROLE_USER
ROLE_SUPER_ADMIN: [ROLE_ADMIN, ROLE_ALLOWED_TO_SWITCH]
具有 ROLE_ADMIN
角色的用户也将拥有 ROLE_USER
角色。具有 ROLE_SUPER_ADMIN
的用户将自动拥有 ROLE_ADMIN
、ROLE_ALLOWED_TO_SWITCH
和 ROLE_USER
(从 ROLE_ADMIN
继承)。
警告
为了使角色层次结构起作用,请不要手动使用 $user->getRoles()
。例如,在从 基础控制器 扩展的控制器中
1 2 3 4 5 6
// BAD - $user->getRoles() will not know about the role hierarchy
$hasAccess = in_array('ROLE_ADMIN', $user->getRoles());
// GOOD - use of the normal security methods
$hasAccess = $this->isGranted('ROLE_ADMIN');
$this->denyAccessUnlessGranted('ROLE_ADMIN');
注意
role_hierarchy
值是静态的 - 例如,您不能将角色层次结构存储在数据库中。如果您需要这样做,请创建一个自定义的 安全投票器,在数据库中查找用户角色。
添加代码以拒绝访问
有两种方式来拒绝访问某些内容
- security.yaml 中的 access_control 允许您保护 URL 模式(例如
/admin/*
)。更简单,但灵活性较差; - 在您的控制器(或其他代码)中.
保护 URL 模式 (access_control)
保护应用程序部分的最基本方法是在 security.yaml
中保护整个 URL 模式。例如,要对所有以 /admin
开头的 URL 要求 ROLE_ADMIN
,您可以
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
# config/packages/security.yaml
security:
# ...
firewalls:
# ...
main:
# ...
access_control:
# require ROLE_ADMIN for /admin*
- { path: '^/admin', roles: ROLE_ADMIN }
# or require ROLE_ADMIN or IS_AUTHENTICATED_FULLY for /admin*
- { path: '^/admin', roles: [IS_AUTHENTICATED_FULLY, ROLE_ADMIN] }
# the 'path' value can be any valid regular expression
# (this one will match URLs like /api/post/7298 and /api/comment/528491)
- { path: ^/api/(post|comment)/\d+$, roles: ROLE_USER }
您可以根据需要定义任意数量的 URL 模式 - 每个模式都是一个正则表达式。但是,每个请求只会匹配一个模式:Symfony 从列表顶部开始,并在找到第一个匹配项时停止
1 2 3 4 5 6 7 8 9 10
# config/packages/security.yaml
security:
# ...
access_control:
# matches /admin/users/*
- { path: '^/admin/users', roles: ROLE_SUPER_ADMIN }
# matches /admin/* except for anything matching the above rule
- { path: '^/admin', roles: ROLE_ADMIN }
在路径前面加上 ^
意味着只有以该模式开头的 URL 才会被匹配。例如,/admin
的路径(不带 ^
)将匹配 /admin/foo
,但也会匹配像 /foo/admin
这样的 URL。
每个 access_control
还可以匹配 IP 地址、主机名和 HTTP 方法。它也可以用于将用户重定向到 URL 模式的 https
版本。对于更复杂的需求,您还可以使用实现 RequestMatcherInterface
的服务。
保护控制器和其他代码
您可以从控制器内部拒绝访问
1 2 3 4 5 6 7 8 9 10
// src/Controller/AdminController.php
// ...
public function adminDashboard(): Response
{
$this->denyAccessUnlessGranted('ROLE_ADMIN');
// or add an optional message - seen by developers
$this->denyAccessUnlessGranted('ROLE_ADMIN', null, 'User tried to access a page without having ROLE_ADMIN');
}
就是这样!如果未授予访问权限,则会抛出一个特殊的 AccessDeniedException,并且控制器中不再调用任何代码。然后,会发生以下两种情况之一
- 如果用户尚未登录,他们将被要求登录(例如,重定向到登录页面)。
- 如果用户已登录,但没有
ROLE_ADMIN
角色,他们将看到 403 访问被拒绝页面(您可以 自定义 该页面)。
保护一个或多个控制器操作的另一种方法是使用 #[IsGranted]
属性。在以下示例中,所有控制器操作都需要 ROLE_ADMIN
权限,但 adminDashboard()
除外,它将需要 ROLE_SUPER_ADMIN
权限
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
// src/Controller/AdminController.php
// ...
use Symfony\Component\Security\Http\Attribute\IsGranted;
#[IsGranted('ROLE_ADMIN')]
class AdminController extends AbstractController
{
// Optionally, you can set a custom message that will be displayed to the user
#[IsGranted('ROLE_SUPER_ADMIN', message: 'You are not allowed to access the admin dashboard.')]
public function adminDashboard(): Response
{
// ...
}
}
如果您想使用自定义状态代码而不是默认代码(即 403),可以通过设置 statusCode
参数来完成
1 2 3 4 5 6 7 8 9 10
// src/Controller/AdminController.php
// ...
use Symfony\Component\Security\Http\Attribute\IsGranted;
#[IsGranted('ROLE_ADMIN', statusCode: 423)]
class AdminController extends AbstractController
{
// ...
}
您还可以使用 exceptionCode
参数设置抛出的 AccessDeniedException 的内部异常代码
1 2 3 4 5 6 7 8 9 10
// src/Controller/AdminController.php
// ...
use Symfony\Component\Security\Http\Attribute\IsGranted;
#[IsGranted('ROLE_ADMIN', statusCode: 403, exceptionCode: 10010)]
class AdminController extends AbstractController
{
// ...
}
模板中的访问控制
如果您想检查当前用户是否具有某个角色,您可以在任何 Twig 模板中使用内置的 is_granted()
助手函数
1 2 3
{% if is_granted('ROLE_ADMIN') %}
<a href="...">Delete</a>
{% endif %}
保护其他服务
您可以通过注入 Security
服务在代码中的任何位置检查访问权限。例如,假设您有一个 SalesReportManager
服务,并且您只想为具有 ROLE_SALES_ADMIN
角色的用户包含额外的详细信息
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
// src/SalesReport/SalesReportManager.php
// ...
use Symfony\Component\Security\Core\Exception\AccessDeniedException;
+ use Symfony\Bundle\SecurityBundle\Security;
class SalesReportManager
{
+ public function __construct(
+ private Security $security,
+ ) {
+ }
public function generateReport(): void
{
$salesData = [];
+ if ($this->security->isGranted('ROLE_SALES_ADMIN')) {
+ $salesData['top_secret_numbers'] = rand();
+ }
// ...
}
// ...
}
如果您正在使用 默认的 services.yaml 配置,则由于自动装配和 Security
类型提示,Symfony 将自动将 security.helper
传递给您的服务。
您还可以使用更底层的 AuthorizationCheckerInterface 服务。它的作用与 Security
相同,但允许您类型提示更具体的接口。
允许非安全访问(即匿名用户)
当访问者尚未登录您的网站时,他们被视为“未经身份验证”,并且没有任何角色。如果您定义了 access_control
规则,这将阻止他们访问您的页面。
在 access_control
配置中,您可以使用 PUBLIC_ACCESS
安全属性来排除某些路由以进行未经身份验证的访问(例如登录页面)
1 2 3 4 5 6 7 8 9 10
# config/packages/security.yaml
security:
# ...
access_control:
# allow unauthenticated users to access the login form
- { path: ^/admin/login, roles: PUBLIC_ACCESS }
# but require authentication for all other admin routes
- { path: ^/admin, roles: ROLE_ADMIN }
在自定义投票器中授予匿名用户访问权限
如果您正在使用 自定义投票器,则可以通过检查令牌上是否未设置用户来允许匿名用户访问
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
// src/Security/PostVoter.php
namespace App\Security;
// ...
use Symfony\Component\Security\Core\Authentication\Token\TokenInterface;
use Symfony\Component\Security\Core\Authentication\User\UserInterface;
use Symfony\Component\Security\Core\Authorization\Voter\Voter;
class PostVoter extends Voter
{
// ...
protected function voteOnAttribute(string $attribute, $subject, TokenInterface $token): bool
{
// ...
if (!$token->getUser() instanceof UserInterface) {
// the user is not authenticated, e.g. only allow them to
// see public posts
return $subject->isPublic();
}
}
}
设置个人用户权限
大多数应用程序需要更具体的访问规则。例如,用户应该只能编辑他们自己在博客上的评论。投票器允许您编写任何您需要的业务逻辑来确定访问权限。使用这些投票器类似于前面章节中实现的基于角色的访问检查。阅读 如何使用投票器检查用户权限 以了解如何实现您自己的投票器。
检查用户是否已登录
如果您只想检查用户是否已登录(您不关心角色),则可以使用以下两个选项。
首先,如果您已为每个用户赋予 ROLE_USER
,则可以检查该角色。
其次,您可以使用特殊的“属性” IS_AUTHENTICATED
代替角色
1 2 3 4 5 6 7 8
// ...
public function adminDashboard(): Response
{
$this->denyAccessUnlessGranted('IS_AUTHENTICATED');
// ...
}
您可以在使用角色的任何地方使用 IS_AUTHENTICATED
:例如 access_control
或在 Twig 中。
IS_AUTHENTICATED
不是角色,但它有点像角色,并且每个已登录的用户都将拥有此角色。实际上,有一些像这样的特殊属性
了解用户如何从会话中刷新
在每个请求结束时(除非您的防火墙是 stateless
),您的 User
对象被序列化到会话中。在下一个请求开始时,它会被反序列化,然后传递给您的用户提供器以“刷新”它(例如,Doctrine 查询以获取新的用户)。
然后,比较两个 User 对象(来自会话的原始对象和刷新的 User 对象),以查看它们是否“相等”。默认情况下,核心 AbstractToken
类比较 getPassword()
、getSalt()
和 getUserIdentifier()
方法的返回值。如果其中任何一个不同,您的用户将被注销。这是一种安全措施,以确保在核心用户数据更改时可以取消恶意用户的身份验证。
但是,在某些情况下,此过程可能会导致意外的身份验证问题。如果您在身份验证方面遇到问题,可能是您确实成功通过了身份验证,但在第一次重定向后立即失去了身份验证。
在这种情况下,请查看用户类上的序列化逻辑(例如 __serialize()
或 serialize()
方法)(如果您有任何逻辑),以确保所有必要的字段都被序列化,并排除所有不必要的字段(例如 Doctrine 关系)。
使用 EquatableInterface 手动比较用户
或者,如果您需要更多地控制“比较用户”过程,请使您的 User 类实现 EquatableInterface。然后,在比较用户时将调用您的 isEqualTo()
方法,而不是核心逻辑。
安全事件
在身份验证过程中,会分发多个事件,允许您挂钩到该过程或自定义发送回用户的响应。您可以通过为这些事件创建一个 事件监听器或订阅者 来做到这一点。
提示
每个安全防火墙都有自己的事件调度器(security.event_dispatcher.FIREWALLNAME
)。事件在全局和防火墙特定的调度器上分发。如果您希望您的监听器仅针对特定防火墙调用,则可以在防火墙调度器上注册。例如,如果您有 api
和 main
防火墙,请使用此配置仅在 main
防火墙中的注销事件上注册
1 2 3 4 5 6 7 8
# config/services.yaml
services:
# ...
App\EventListener\LogoutSubscriber:
tags:
- name: kernel.event_subscriber
dispatcher: security.event_dispatcher.main
身份验证事件
- CheckPassportEvent
- 在验证器创建 安全 passport 后分发。此事件的监听器执行实际的身份验证检查(例如检查 passport、验证 CSRF 令牌等)
- AuthenticationTokenCreatedEvent
- 在 passport 经过验证且验证器创建安全令牌(和用户)后分发。这可以在高级用例中使用,在这些用例中,您需要修改创建的令牌(例如,用于多因素身份验证)。
- AuthenticationSuccessEvent
- 在身份验证即将成功时分发。这是最后一个可以通过抛出
AuthenticationException
来使身份验证失败的事件。 - LoginSuccessEvent
- 在身份验证完全成功后分发。此事件的监听器可以修改发送回用户的响应。
- LoginFailureEvent
- 在身份验证期间抛出
AuthenticationException
后分发。此事件的监听器可以修改发送回用户的错误响应。
其他事件
- InteractiveLoginEvent
- 仅当验证器实现 InteractiveAuthenticatorInterface 时分发,这表明登录需要显式的用户操作(例如登录表单)。此事件的监听器可以修改发送回用户的响应。
- LogoutEvent
- 在用户注销您的应用程序之前分发。请参阅 安全。
- TokenDeauthenticatedEvent
- 当用户被取消身份验证时分发,例如因为密码已更改。请参阅 安全。
- SwitchUserEvent
- 在模拟完成后分发。请参阅 如何模拟用户。
常见问题
- 我可以有多个防火墙吗?
- 是的!但是,每个防火墙都像一个单独的安全系统:在一个防火墙中通过身份验证并不会使您在另一个防火墙中通过身份验证。每个防火墙可以有多种允许身份验证的方式(例如,表单登录和 API 密钥身份验证)。如果您想在防火墙之间共享身份验证,则必须为不同的防火墙显式指定相同的 安全配置参考 (SecurityBundle)。
- 安全似乎在我的错误页面上不起作用
- 由于路由在安全之前完成,因此 404 错误页面不受任何防火墙的覆盖。这意味着您无法在这些页面上检查安全性,甚至无法访问用户对象。有关更多详细信息,请参阅 如何自定义错误页面。
- 我的身份验证似乎不起作用:没有错误,但我永远不会登录
- 有时身份验证可能成功,但在重定向后,由于从会话加载
User
对象时出现问题,您会立即注销。要查看这是否是一个问题,请检查您的日志文件(var/log/dev.log
)以查找日志消息。 - 由于用户已更改,无法刷新令牌
- 如果您看到此消息,则可能有两种可能的原因。首先,从会话加载 User 可能存在问题。请参阅 安全。其次,如果自上次页面刷新以来数据库中某些用户信息已更改,Symfony 将出于安全原因有意注销用户。