创建和编辑对象
本文档将介绍创建和编辑操作。它将介绍这些视图中可用的字段和表单的配置以及任何其他相关设置。
基本配置
可能影响创建或编辑视图的 SonataAdmin 选项
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
# config/packages/sonata_admin.yaml
sonata_admin:
options:
html5_validate: true # enable or disable html5 form validation
confirm_exit: true # enable or disable a confirmation before navigating away
js_debug: false # enable or disable to show javascript debug messages
use_select2: true # enable or disable usage of the Select2 jQuery library
use_icheck: true # enable or disable usage of the iCheck library
use_bootlint: false # enable or disable usage of Bootlint
use_stickyforms: true # enable or disable the floating buttons
form_type: standard # can also be 'horizontal'
templates:
edit: '@SonataAdmin/CRUD/edit.html.twig'
tab_menu_template: '@SonataAdmin/Core/tab_menu_template.html.twig'
有关可选库的更多信息
- Select2: https://github.com/select2/select2
- iCheck: http://icheck.fronteed.com/
- Bootlint: https://github.com/twbs/bootlint#in-the-browser
路由
您可以通过删除 Admin 中相应的路由来禁用创建或编辑实体。有关路由的更多详细信息,请参阅路由
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
// src/Admin/PersonAdmin.php
final class PersonAdmin extends AbstractAdmin
{
protected function configureRoutes(RouteCollectionInterface $collection): void
{
/* Removing the edit route will disable editing entities. It will also
use the 'show' view as default link on the identifier columns in the list view. */
$collection->remove('edit');
/* Removing the create route will disable creating new entities. It will also
remove the 'Add new' button in the list view. */
$collection->remove('create');
}
}
添加表单字段
在 configureFormFields
方法中,您可以定义在编辑或创建实体时应显示的字段。每个字段都必须添加到特定的表单组。表单组可以选择添加到选项卡。有关配置表单组的更多信息,请参阅FormGroup 选项
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
// src/Admin/PersonAdmin.php
final class PersonAdmin extends AbstractAdmin
{
protected function configureFormFields(FormMapper $form): void
{
$form
->tab('General') // The tab call is optional
->with('Addresses')
->add('title') // Add a field and let Sonata decide which type to use
->add('streetname', TextType::class) // Add a textfield
->add('housenumber', NumberType::class) // Add a number field
->add('housenumberAddition', TextType::class, ['required' => false]) // Add a non-required text field
->end() // End form group
->end() // End tab
;
}
}
使用 FormMapper add 方法,您可以添加表单字段。add 方法有 4 个参数
name
:您的实体的名称。type
:要显示的字段类型;默认情况下,这是null
,让 Sonata 决定使用哪种类型。有关可用类型的更多信息,请参阅字段类型。options
:用于该字段的表单选项。这些选项可能因类型而异。有关可用选项的更多信息,请参阅字段类型。fieldDescriptionOptions
:字段描述选项。此处的选项会传递到字段模板。有关更多信息,请参阅表单类型,FieldDescription 选项。
注意
在 name
中输入的属性应通过 getter/setter 或公共访问在您的实体中可用。
FormGroup 选项
当向您的编辑/创建表单添加表单组时,您可以为该组本身指定一些选项。
collapsed
:目前未使用class
:您的表单组在 admin 中的类;默认情况下,该值设置为col-md-12
。fields
:您的表单组中的字段(除非您知道自己在做什么,否则不应覆盖此项)。box_class
:您的表单组框在 admin 中的类;默认情况下,该值设置为box box-primary
。description
:显示在表单组顶部的文本。translation_domain
:表单组标题的翻译域(默认情况下使用 Admin 翻译域)。
要指定选项,请执行以下操作
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
// src/Admin/PersonAdmin.php
final class PersonAdmin extends AbstractAdmin
{
protected function configureFormFields(FormMapper $form): void
{
$form
->tab('General') // the tab call is optional
->with('Addresses', [
'class' => 'col-md-8',
'box_class' => 'box box-solid box-danger',
'description' => 'Lorem ipsum',
// ...
])
->add('title')
// ...
->end()
->end()
;
}
}
这是一个关于如何自定义组的 box_class 的示例
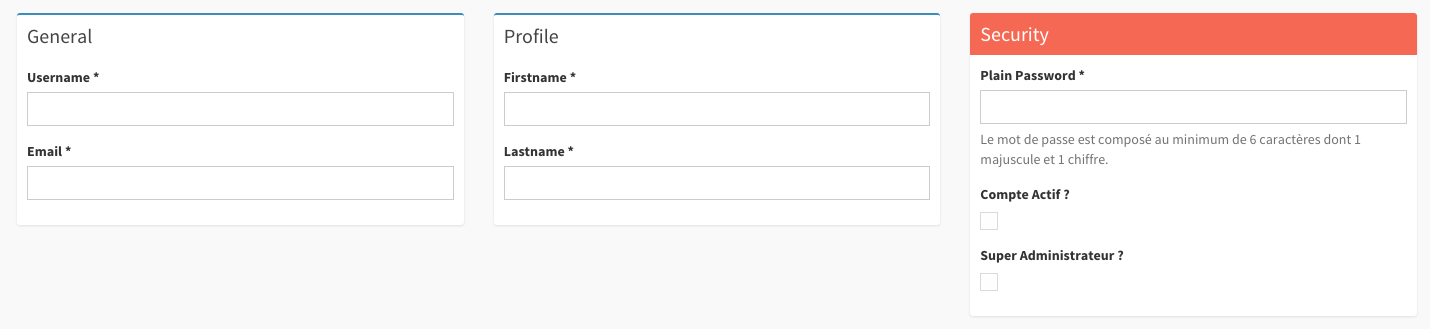
显示自定义数据/模板
如果您需要在某些字段之间进行特定布局,您可以使用 sonata TemplateType 定义自定义模板
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
namespace App\Admin;
use Sonata\AdminBundle\Admin\AbstractAdmin;
use Sonata\AdminBundle\Form\FormMapper;
use Sonata\AdminBundle\Form\Type\TemplateType;
final class PersonAdmin extends AbstractAdmin
{
protected function configureFormFields(FormMapper $form): void
{
$form
->add('title')
->add('googleMap', TemplateType::class, [
'template' => 'path/to/your/template.html.twig'
'parameters' => [
'url' => $this->generateGoogleMapUrl($this->getSubject()),
],
])
->add('streetname', TextType::class)
->add('housenumber', NumberType::class);
}
}
相关模板
1
<a href="{{ url }}">{{ object.title }}</a>
嵌入其他 Admin
注意
待办事项:如何在另一个 Admin 中嵌入一个 Admin(1:1、1:M、M:M) 如何从嵌入式 Admin 的代码中访问正确的对象
本作品,包括代码示例,根据 Creative Commons BY-SA 3.0 许可获得许可。